Django Introduction¶
In this initial project, we will be introduced to formal use of the shell to create our first Django Project. As discussed, if you are using a Mac, you have a terminal available by looking in the search bar. The terminal is a place to interact with and create and edit programs. We will use the following commands:
cd
(change directory)pwd
(print working directory)ls
(list files)mkdir
(make directory)touch
(create file)
For example, I have a folder on my desktop where I keep all my files for this semester. I can open a new terminal and type
cd Desktop spring_18
and I will now be located in this folder. If I wanted to see the files here, I can write
ls -F
where the -F
flags directories.
If we wanted to make a new directory named images
, we can use
mkdir images
To create a new file, for example home.html
, we would use
touch home.html
Finally, we will be using virtual environments for this project and will
use pipenv
to do this. In our terminal we can type
pip install pipenv
A First Django Project¶
To begin, we will create an empty project with Django to get a feel for using the virtual environment in the shell. We need to check that we have git working, and that we can open SublimeText (or another text editor) on our computers.
Set up Directory and Virtual Environment¶
Let’s create a folder for our project on our desktop called django, and navigate into this folder by typing:
mkdir django
cd django
Now we will create a virtual environment where we install django.
pipenv install django
and activate it with
pipenv shell
Start a Django Project¶
Now, we can start a project to test our new django installation. Let’s
create a project called mysite
by typing the following in the
terminal:
django-admin startproject mysite .
We can now examine the structure of the directory we have created with
tree
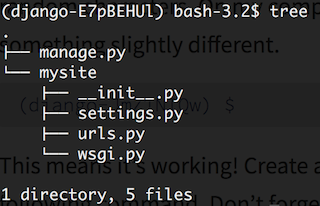
This is the standard django project structure. A manage.py
python
file, and a directory named mysite
containing four files:
__init__.py
, settings.py
, urls.py
, and wsgi.py
. To see
the blank project in action, we will use the built in server, located in
the manage.py
file. To use this, we write
python manage.py runserver
We should see the project launched on our local computer at http://127.0.0.1:8000/. When we go to this page, we should see the following:
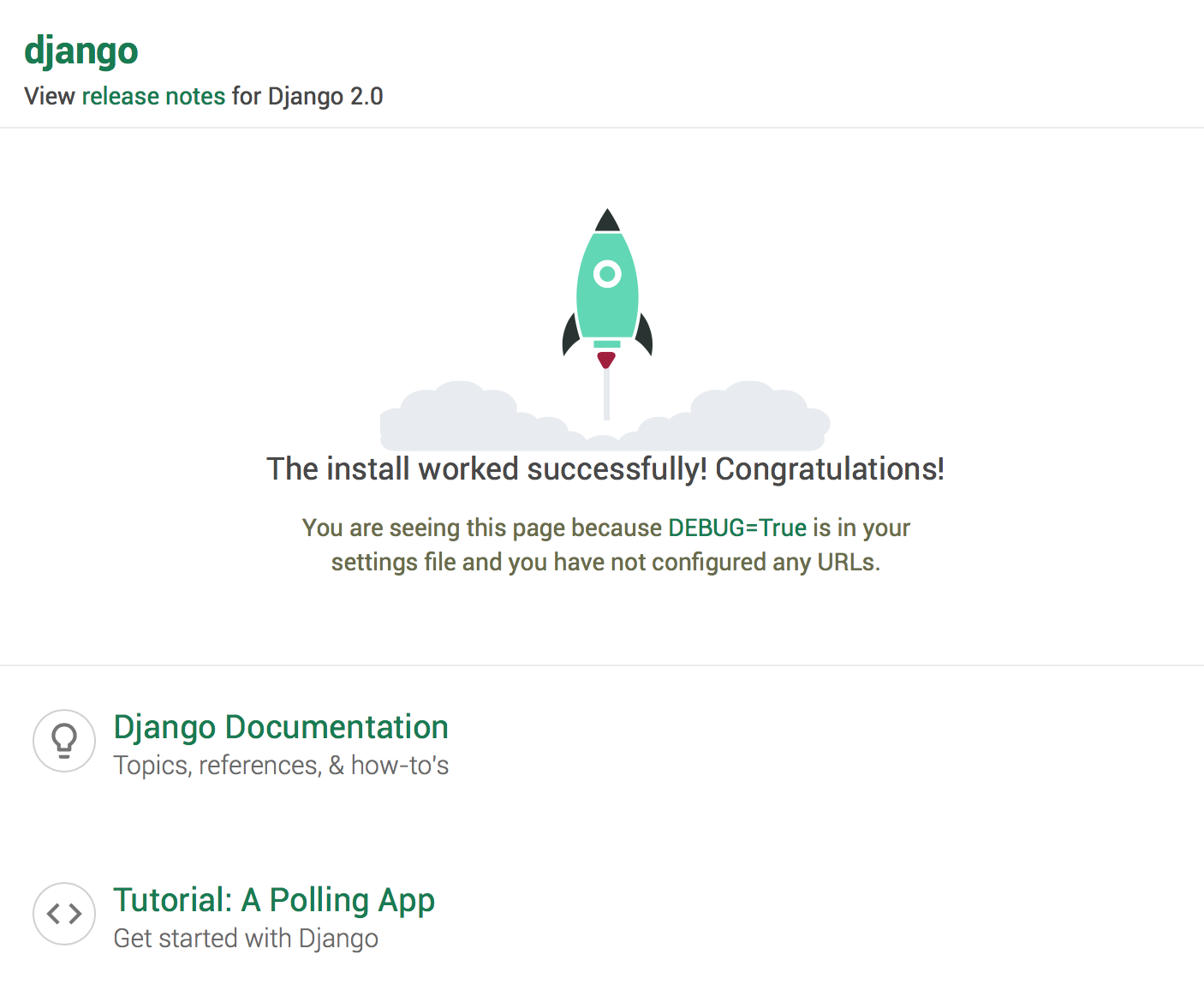
Now that we’ve started our project, we will add some content to it.
Starting an App¶
Similar to how we made use of the default Django project structure, within our project we will create an app named pages with the command
python manage.py startapp pages
Now, we have a directory with the following structure
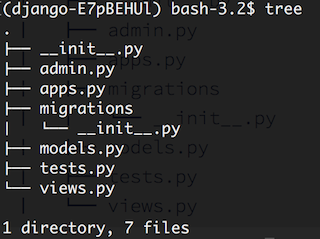
We now will link this application to the Django project by opening the
settings.py
file located in the main mysite
directory in a text
editor. Find INSTALLED_APPS
, and we will add our app pages
to
the list as shown.
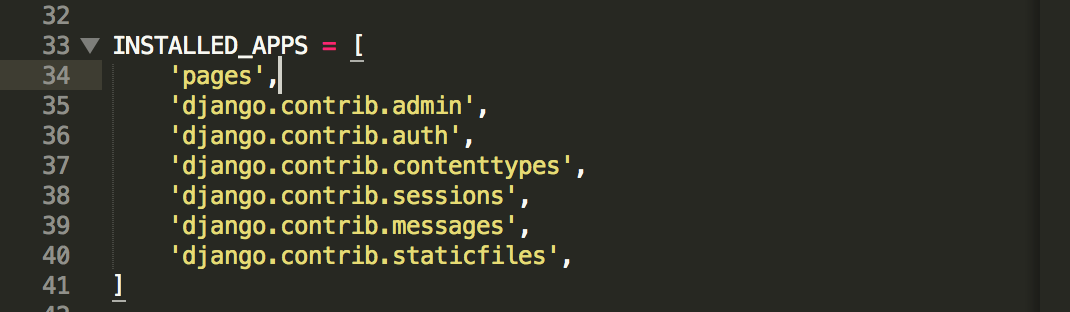
Django Views¶
Now, we want to add some content to our app, and establish some connections that allow the content to be seen. In Django, the views determing the content displayed. We then have to use the urlconfs to decide where the content goes.
Starting with the views file, lets add the following code:
from django.shortcuts import render
from django.http import HttpResponse
# Create your views here.
def homepageview(request):
return HttpResponse("<h3>It's So Wonderful to see you Jacob!</h3>")
This view will accept a request, and return the HTML header that I’ve
placed in HttpResponse()
. Now, we have to establish the location for
the file using a urls
file. We create a new file in our pages
directory named urls.py
. Here, will use the urlpatterns call and
provide a path to our page. If we want it to be at a page called
home
, we could write the following:
from django.urls import path
from . import views
urlpatterns = [
path('', views.homepageview, name = 'home')
]
This establishes the link within the application, and we need to connect
this to the larger project within the base urls.py
file. This was
already created with our project, and we want it to read as follows:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('pages.urls')),
]
Now, if we run our server again and navigate to http://127.0.0.1:8000/ we should see the results of our work.
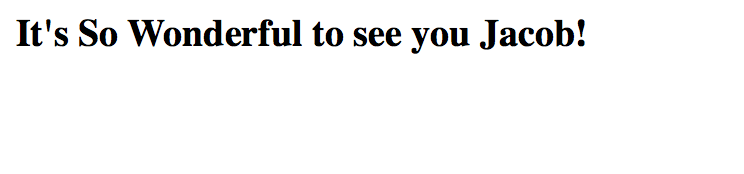